A Tiled Island Map
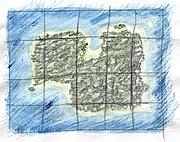
Smooth coastline!
For the island game I’m creating using App Engine, I need a tiled map. I wasn’t expecting this to be difficult, but the sheer number of different tiles required is daunting. If I limit roads to only entering a tile from the north, south, east, or west, there are still 15 ways for roads to cross a tile. Since I don’t want blocky, square islands with abrupt land/ocean transitions, I need a set of 46 coastline tiles. Start multiplying by different types of base tile (forests, fields, mountains, tiny villages, big cities, etc.) and the situation quickly gets out of hand.
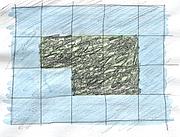
Blocky coastline
The only sane solution appears to be transparent overlays which are composited on demand. I don’t see a way to do server-side compositing with App Engine (plus I don’t think it would scale well), so that leaves client-side compositing. I did a simple mock-up with transparent PNG images, using absolute positioning to stack them on top of each other. It seems workable. Traditionally, transparent PNG images weren’t supported well by IE6, but since only 10% of the visitors to my site are using IE6, I might just forget about supporting it.
So that’s the long-term plan. In the spirit of getting a working prototype as quickly as possible, though, I’m going to start with a really simple tileset where I can pre-generate all the combinations and just serve static images. Here are the 6 tiles I’m starting with:

Ocean

Land

Mountains

City

Field

Road
I’m not going to allow roads over mountains for now, so there are 50 possible tiles (16 road combinations * (city, field, land) + ocean + mountains). Pre-generating all the combinations is a snap using PIL.
#!/usr/bin/env python2.5
import os
from PIL import Image
def composite(images):
"""Composite a stack of images, [0] on top, [-1] on bottom."""
top = images[0]
for lower in images[1:]:
top = Image.composite(top, lower, top)
return top
def combinations(list):
"""Generate all combinations of items in list."""
if list:
for c in combinations(list[:-1]):
yield c
yield c + [list[-1]]
else:
yield []
def load(name):
return Image.open(os.path.join('sources', '%s.png' % name))
def save(name, image):
image.save(os.path.join('img', '%s.png' % name))
def make_roads(name, above, below):
"""Save tiles with roads. Images in above will be composited above
the roads. Images in below will be composited below the roads."""
roads = dict(w=load('road_overlay'),
s=load('road_overlay').transpose(Image.ROTATE_90),
e=load('road_overlay').transpose(Image.ROTATE_180),
n=load('road_overlay').transpose(Image.ROTATE_270))
above = [load(filename) for filename in above]
below = [load(filename) for filename in below]
for directions in combinations(sorted(roads.keys())):
out = composite(above + [roads[x] for x in directions] + below)
if directions:
save('%s_road_%s' % (name, ''.join(directions)), out)
else:
save(name, out)
def main():
save('mountains', load('mountains'))
save('ocean', load('ocean'))
make_roads('land', [], ['land'])
make_roads('city', ['city_overlay'], ['land'])
make_roads('field', [], ['field_overlay', 'land'])
if __name__ == '__main__':
main()
PIL also comes in handy to paste together the first proof-of-concept map using the new tiles:
#!/usr/bin/env python2.5
import os
import sys
from PIL import Image
TILE_SIZE = 50 # Width/height of a tile in pixels.
in_file, out_file = sys.argv[1:3]
data = [line.split() for line in open(in_file).readlines()]
map = Image.new('RGB', (TILE_SIZE * len(data[0]), TILE_SIZE * len(data)))
for y, row in enumerate(data):
for x, name in enumerate(row):
image = Image.open(os.path.join('img', '%s.png' % name))
map.paste(image, (x * TILE_SIZE, y * TILE_SIZE))
map.save(out_file)
And here it is: The first example map!
Next on the agenda: Getting Django running on App Engine and serving this example map using HTML.